This technical paper guides developers through the detailed process of integrating #RedStone Oracles into a decentralized application.
This document will cover the setup, integration, and testing phases, ensuring developers can harness these capabilities within their DApps.
1. Introduction
RedStone Oracles offer a decentralized solution for accessing real-time data within blockchain applications. They utilize a network of data providers and the Arweave blockchain for data storage, ensuring both integrity and permanence. This guide will detail the steps necessary to integrate these oracles, from initial installation to advanced customization and testing.
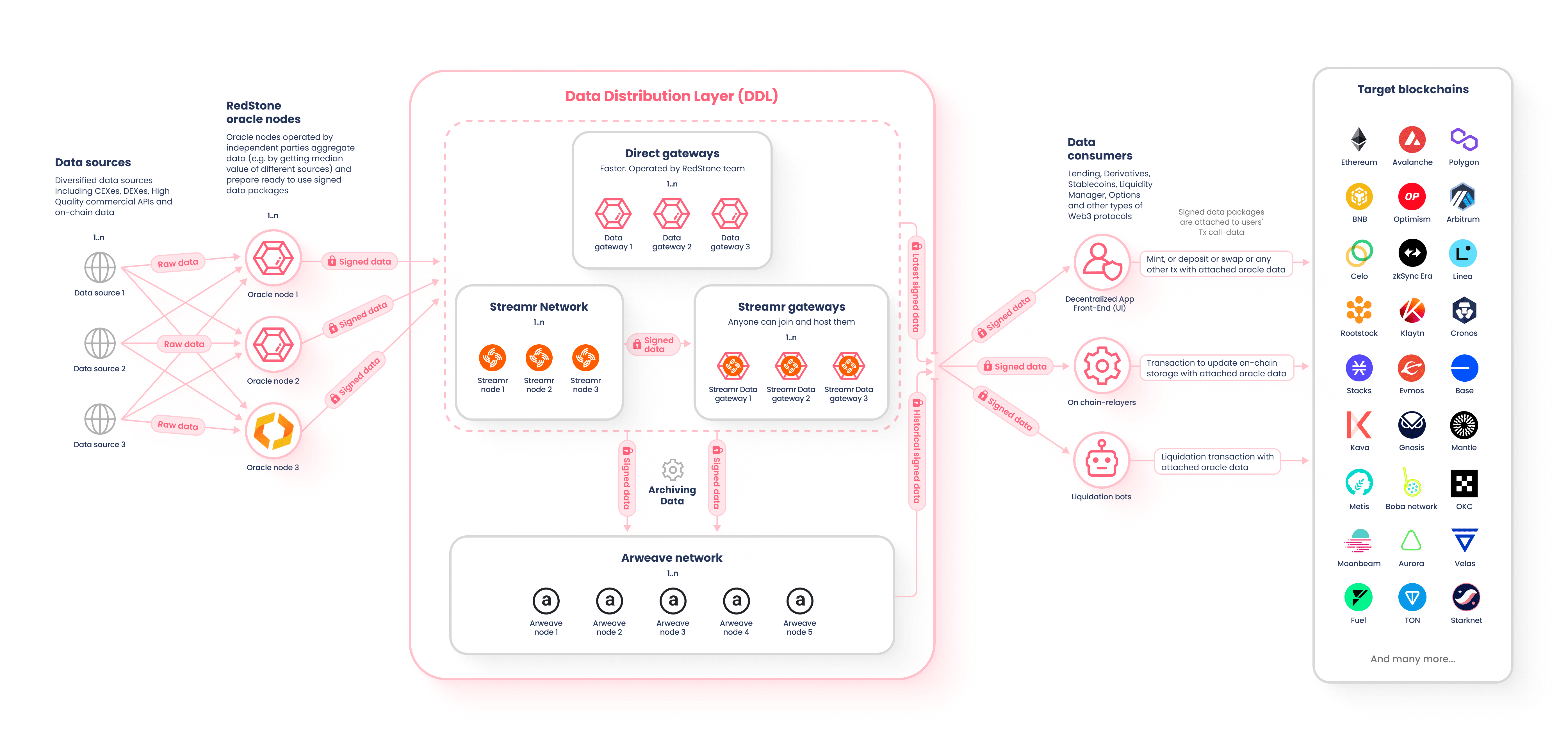
2. Setup Requirements
Before starting the integration process, ensure the following prerequisites are met:
Node.js and npm installed on your development machine.
A basic understanding of Solidity and smart contracts.
Familiarity with JavaScript and #Ethereum development tools like Hardhat or Foundry.
3. Installation
Node Package Installation:
npm install @redstone-finance/evm-connector
Foundry Setup:
Foundry users need to install dependencies through git submodules:forge install redstone-finance/redstone-oracles-monorepo
forge install OpenZeppelin/openzeppelin-contracts@v4.9.5Updating Remappings in Foundry:
Add paths to the remappings.txt:echo "@redstone-finance/evm-connector/dist/contracts/=lib/redstone-oracles-monorepo/packages/evm-connector/contracts/
@openzeppelin/contracts=lib/openzeppelin-contracts/contracts/" >> remappings.txt
4. Smart Contract Integration
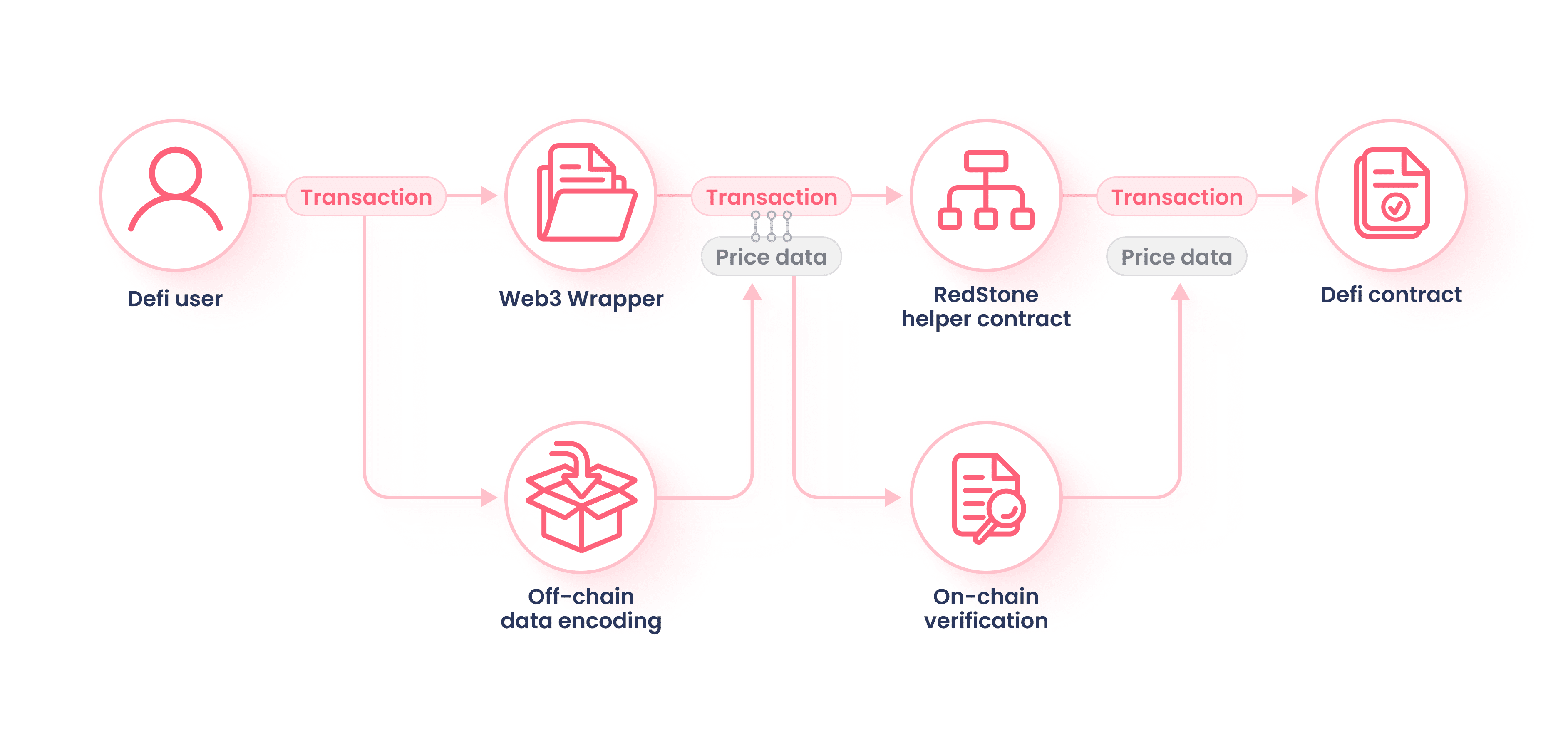
Inherit from Base Contracts:
Your contract should extend from one of RedStone's base contracts to utilize oracle data:import "@redstone-finance/evm-connector/contracts/data-services/MainDemoConsumerBase.sol";
contract YourContractName extends MainDemoConsumerBase {
// Additional contract code here
}Fetching Data:
Implement functions to fetch and handle oracle data within your contract:function fetchOracleData(bytes32 dataIdentifier) public returns (uint256) {
uint256 dataValue = getOracleNumericValueFromTxMsg(dataIdentifier);
return dataValue;
}
5. Front-End Integration
Setting Up the Ethers.js Wrapper:
Integrate the RedStone EVM connector with ethers.js to interact with the smart contract:const { WrapperBuilder } = require("@redstone-finance/evm-connector");
const ethers = require("ethers");
let provider = new ethers.providers.JsonRpcProvider();
let contract = new ethers.Contract(contractAddress, contractABI, provider);
let wrappedContract = WrapperBuilder.wrap(contract).usingDataService({
dataFeeds: ["ETH", "BTC"]
});
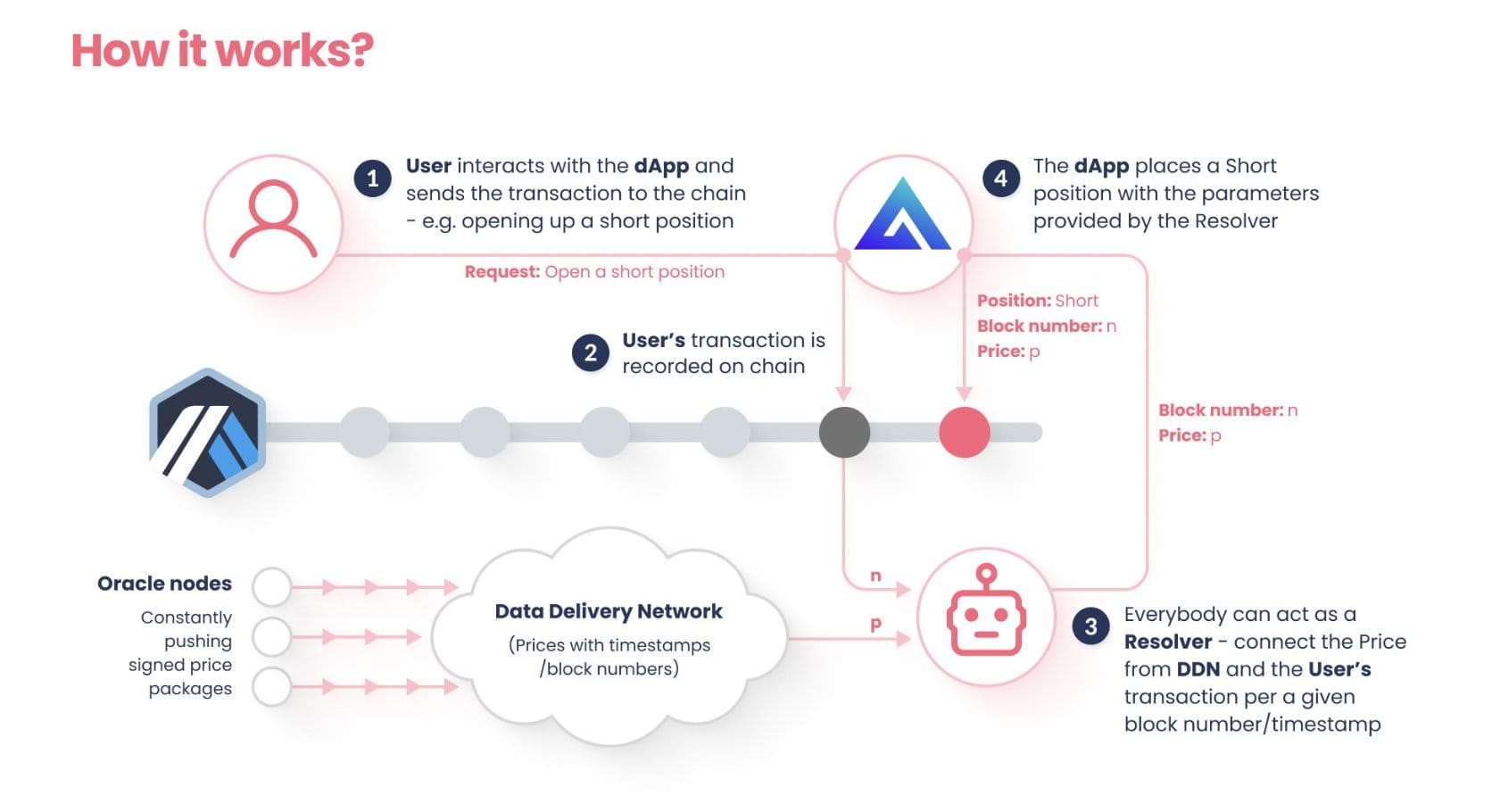
Interacting with Contracts:
Use the wrapped contract to call functions as you would normally:async function callContractFunction() {
let result = await wrappedContract.fetchOracleData("ETH");
console.log(`Oracle Data: ${result}`);
}
6. Testing and Deployment
Hardhat Testing:
Use Hardhat to simulate interactions and test the contract's response to oracle data:const { expect } = require("chai");
describe("Oracle Integration Tests", function () {
it("should fetch oracle data correctly", async function () {
const data = await wrappedContract.fetchOracleData("ETH");
expect(data).to.be.a('number');
});
});Deployment:
Deploy your contracts to a live network after thorough testing:truffle migrate --network mainnet
7. Conclusion
Integrating RedStone Oracles into your DApp significantly enhances its capability to interact with real-time data efficiently and securely. By following this step-by-step guide, developers can ensure a robust integration, leveraging RedStone's advanced blockchain-based oracle solutions for kickstarting decentralized applications.